I didn't see a tutorial for a quest page ANYWHERE, so I decided to make one myself. This took FOREVER, so I hope it works for you!
First things first, let's create a couple new columns in the database, namely questsdone and questday under [prefix]_users.
You want both to be set to int with the length set to 100 and with a default value of 0. (You don't technically need a length of 100 for questday, but you need at least 2. I'd go higher to be on the safe side though.) Here's an example of questsdone, but you set up both the same way.
Next, we need to set up a new file called questscontroller.php under controller/main. (You can name it anything, but it will be found at yourURL/quests in my example.)
It should look like this.
Now we need to set up the other half of the webpage. Go to view/main and make a file called questsview.php. If you used a different name, make sure this file matches. Begin by adding this code.
I will finish this in a reply when I get out of class!
First things first, let's create a couple new columns in the database, namely questsdone and questday under [prefix]_users.
You want both to be set to int with the length set to 100 and with a default value of 0. (You don't technically need a length of 100 for questday, but you need at least 2. I'd go higher to be on the safe side though.) Here's an example of questsdone, but you set up both the same way.
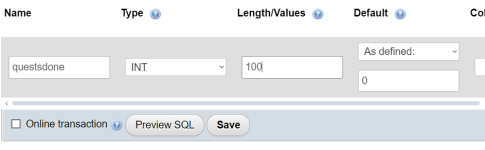
Next, we need to set up a new file called questscontroller.php under controller/main. (You can name it anything, but it will be found at yourURL/quests in my example.)
It should look like this.
PHP:
<?php
namespace Controller\Main;
use Resource\Core\AppController;
use Resource\Core\Registry;
class QuestsController extends AppController{
//You need to name the controller the same name as your file, but make sure the first word and "controller" are both capitalized where it says
//"class QuestsController"
public function __construct(){
//This means only members can access the page.
parent::__construct("member");
}
public function index(){
$mysidia = Registry::get("mysidia");
}
}
Now we need to set up the other half of the webpage. Go to view/main and make a file called questsview.php. If you used a different name, make sure this file matches. Begin by adding this code.
PHP:
<?php
namespace View\Main;
use Resource\Core\Registry;
use Resource\Core\View;
use Resource\Core\Model;
//I'm not going to lie, I just covered all my bases with the "use" things below.
//They allow you to call certain variables, and make different containers or components.
use Model\DomainModel\Member;
use Model\DomainModel\User;
use Model\DomainModel\UserProfile;
use Model\DomainModel\Adoptable;
use Model\DomainModel\OwnedAdoptable;
use Model\DomainModel\Item;
use Model\DomainModel\OwnedItem;
use Resource\GUI\Document\Comment;
use Resource\GUI\Component\Link;
use Resource\GUI\Component\Image;
use Resource\GUI\Container\Form;
use Resource\GUI\Component\Button;
class QuestsView extends View{
//Make sure, yet again, that the first word and "View" are both capitalized.
//Also make sure it matches your filename.
public function index(){
$mysidia = Registry::get("mysidia");
$document = $this->document;
$document->setTitle("Quest Board");
//Title the page whatever you like. This will be the header for the page.
}
}
I will finish this in a reply when I get out of class!
Last edited: