Hello~ I finally finished porting IntoRain's News System over to 1.3.6! I have attached a .zip that has the main files so you can drop them into your site. Nothing should be overwritten.
Step 1 - Prepare the Database
Go to your PHP Myadmin and add 2 new database tables. Remember to use the prefix that your site uses (if you kept the default it will be 'adopts_'). The pictures below should show what you need to add.
adopts_news :
adopts_newscomments :
Step 2 - Preparing the Model Files
Next step is to open your site files. Go to model/domainmodel and create 2 new files. news.php and newscomments.php.
news.php:
newscomments.php:
Step 3 - Preparing the Controller
Next, open your controller/main folder, and add a new newscontroller.php file.
newscontroller.php:
Step 4 - Preparing the View
Next, open your view/main folder, and add a new newsview.php file.
newsview.php:
That should allow you to view news on your site, read/add comments, etc. Now we need to add AdminCP functions!
Step 1 - Prepare the Database
Go to your PHP Myadmin and add 2 new database tables. Remember to use the prefix that your site uses (if you kept the default it will be 'adopts_'). The pictures below should show what you need to add.
adopts_news :
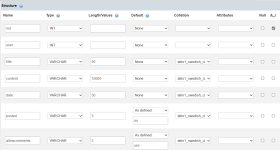
adopts_newscomments :
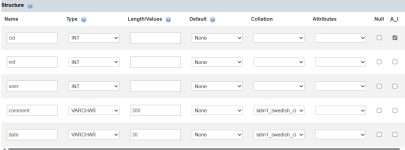
Step 2 - Preparing the Model Files
Next step is to open your site files. Go to model/domainmodel and create 2 new files. news.php and newscomments.php.
news.php:
PHP:
<?php
namespace Model\DomainModel;
use DateTime;
use HTMLPurifier;
use Resource\Core\Model;
use Resource\Core\Registry;
use Resource\Exception\InvalidIDException;
use Resource\Utility\Date;
use Resource\Collection\ArrayList;
class News extends Model{
protected $nid;
protected $user;
protected $title;
protected $content;
protected $date;
protected $posted;
protected $allowcomments;
private $htmlPurifier;
public function __construct($nid, $dto = NULL, $htmlPurifier = TRUE){
$mysidia = Registry::get("mysidia");
if($htmlPurifier) $this->htmlPurifier = new HTMLPurifier;
if(!$dto){
$whereClause = "nid = '{$nid}'";
$dto = $mysidia->db->select("news",array(),$whereClause)->fetchObject();
if(!is_object($dto)) throw new InvalidIDException("News {$nid} does not exist...");
}
parent::__construct($dto);
}
protected function createFromDTO($dto){
parent::createFromDTO($dto);
$this->date = new Date($dto->date);
}
public function getID(){
return $this->nid;
}
public function getProfile(){
return new UserProfile($this->user);
}
public function getUserID($fetchMode = ""){
if($fetchMode == Model::MODEL) return new Member($this->user);
else return $this->user;
}
public function getUsername(){
if(!$this->user) return NULL;
return $this->getUserID(Model::MODEL)->getUsername();
}
public function getTitle(){
return $this->title;
}
public function getContent(){
return $this->content;
}
public function getDate($format = NULL){
return $format ? $this->date->format($format) : $this->date;
}
public function getPosted(){
return $this->posted;
}
public function getAllowComment(){
return $this->allowcomments;
}
public function getComments(){
$mysidia = Registry::get("mysidia");
$stmt = $mysidia->db->select("newscomments",array("cid"),"nid = {$this->getID()} ORDER BY date,nid");
$comments = new ArrayList;
while($dto = $stmt->fetchObject()){
$comments->add(new NewsComments($dto->cid, $dto));
}
return $comments;
}
public function addComment($content, $date, $user){
$mysidia = Registry::get("mysidia");
if($this->htmlPurifier) $content = $this->htmlPurifier->purify($this->format($content));
$mysidia->db->insert("newscomments", ["cid" => NULL, "nid" => $this->nid, "user" => $mysidia->user->getID(), "comment" => $content, "date" => $date]);
}
public function format($text){
return stripslashes(html_entity_decode(strip_tags($text, "<strong><em><b><i><u><s><p><sub><sup><a><li><ul><ol>")));
}
public function todayDate($format = NULL){
$dateTime = new DateTime;
$date = $dateTime->format($format);
return $date;
}
public function newDate($date){
$mysidia = Registry::get("mysidia");
$this->date = $date;
$this->save("date", $this->date);
}
public function getCommentNumber(){
$mysidia = Registry::get("mysidia");
$stmt = $mysidia->db->select("newscomments", ["cid"], "nid = '{$this->nid}'");
return $stmt->rowCount();
}
protected function save($field, $value){
$mysidia = Registry::get("mysidia");
$mysidia->db->update("news", [$field => $value], "nid='{$this->nid}'");
}
}
?>
newscomments.php:
PHP:
<?php
namespace Model\DomainModel;
use DateTime;
use Resource\Core\Model;
use Resource\Core\Registry;
use Resource\Exception\InvalidIDException;
use Resource\Utility\Date;
class NewsComments extends Model{
protected $cid;
protected $nid;
protected $user;
protected $comment;
protected $date;
public function __construct($cid, $dto = NULL){
$mysidia = Registry::get("mysidia");
if(!$dto){
$whereClause = "cid = '{$cid}'";
$dto = $mysidia->db->select("newscomments",array(),$whereClause)->fetchObject();
if(!is_object($dto)) throw new InvalidIDException("Comment {$cid} does not exist...");
}
parent::__construct($dto);
}
protected function createFromDTO($dto){
parent::createFromDTO($dto);
$this->date = new Date($dto->date);
}
public function getID(){
return $this->cid;
}
public function getNewsID(){
return $this->nid;
}
public function getProfile(){
return new UserProfile($this->user);
}
public function getUserID($fetchMode = ""){
if($fetchMode == Model::MODEL) return new Member($this->user);
else return $this->user;
}
public function getUsername(){
if(!$this->user) return NULL;
return $this->getUserID(Model::MODEL)->getUsername();
}
public function getComment(){
return $this->comment;
}
public function getDate($format = NULL){
return $format ? $this->date->format($format) : $this->date;
}
public function todayDate(){
$dateTime = new DateTime;
$date = $dateTime->format('Y-m-d H:i:s');
return $date;
}
public function newDate($date){
$mysidia = Registry::get("mysidia");
$this->date = $date;
$this->save("date", $this->date);
}
protected function save($field, $value){
$mysidia = Registry::get("mysidia");
$mysidia->db->update("newscomments", [$field => $value], "cid='{$this->cid}'");
}
}
?>
Step 3 - Preparing the Controller
Next, open your controller/main folder, and add a new newscontroller.php file.
newscontroller.php:
PHP:
<?php
namespace Controller\Main;
use Model\DomainModel\News;
use Model\DomainModel\NewsComments;
use Resource\Core\AppController;
use Resource\Core\Registry;
use Resource\Core\Pagination;
use Resource\Collection\ArrayList;
use Resource\Exception\NoPermissionException;
use Resource\Exception\BlankFieldException;
use Resource\Exception\GuestNoaccessException;
class NewsController extends AppController{
public function __construct(){
parent::__construct("member");
}
public function index(){
$mysidia = Registry::get("mysidia");
$total = $mysidia->db->select("news", ["nid"], "posted='yes'")->rowCount();
if($total == 0) throw new NoPermissionException("There is currently no news to display!");
$pagination = new Pagination($total, $mysidia->settings->pagination,
"news", $mysidia->input->get("page"));
$stmt = $mysidia->db->select("news", [], "posted='yes' ORDER BY nid DESC LIMIT {$pagination->getLimit()},{$pagination->getRowsperPage()}");
$news = new ArrayList;
while($dto = $stmt->fetchObject()){
$news->add(new News($dto->nid, $dto));
}
$this->setField("pagination", $pagination);
$this->setField("news", $news);
}
public function view($nid){
$mysidia = Registry::get("mysidia");
$news = new News($nid);
if($news->getPosted() == "no"){
if($mysidia->usergroup->getpermission("canmanagecontent") != "yes")//rootadmins, admins and artists
{
throw new NoPermissionException("This message doesn't exist or isn't yet viewable.");
}
}
$total = $mysidia->db->select("newscomments",["cid"],"nid = {$news->getID()}")->rowCount();
$pagination = new Pagination($total, $mysidia->settings->pagination,
"news/view/{$news->getID()}", $mysidia->input->get("page"));
$stmt = $mysidia->db->select("newscomments", [], "nid='{$news->getID()}' ORDER BY date DESC LIMIT {$pagination->getLimit()},{$pagination->getRowsperPage()}");
$newscom = new ArrayList;
while($dto = $stmt->fetchObject()){
$newscom->add(new NewsComments($dto->cid, $dto));
}
$this->setField("pagination", $pagination);
$this->setField("news", $news);
$this->setField("newscomments", $newscom);
if($mysidia->input->post("submit")){
if(!$mysidia->input->rawPost("commenttext"))
throw new BlankFieldException("Comment cannot be blank.");
if($mysidia->user->getPermission("canvm") == "no")
throw new NoPermissionException("You don't have permission to post comments.");
if($news->getPosted() == "no")
throw new NoPermissionException("Can't post comments to drafts.");
$count = $mysidia->db->select("newscomments",array("cid"),"comment= '{$mysidia->input->rawPost("commenttext")}' and nid= '{$news->getID()}' and user= '{$mysidia->user->getID()}' LIMIT 1")->rowCount();
if($count != 0)
throw new NoPermissionException("Oops, seems like you tried to post the same comment.");
if($news->getAllowComment() == "no")
throw new NoPermissionException("No comments allowed on this news post.");
if(!$mysidia->user->isLoggedIn())
throw new GuestNoaccessException("guest");
}
}
}
?>
Step 4 - Preparing the View
Next, open your view/main folder, and add a new newsview.php file.
newsview.php:
PHP:
<?php
namespace View\Main;
use Model\DomainModel\News;
use Model\DomainModel\NewsComments;
use Resource\Core\Registry;
use Resource\Core\View;
use Resource\Core\Model;
use Resource\Core\Mysidia;
use Resource\Model\DomainModel;
use Resource\GUI\Document\Comment;
use Resource\GUI\Container\FieldSet;
use Resource\GUI\Container\Form;
use Resource\GUI\Component\Button;
use Service\Builder\CKEditorBuilder;
class NewsView extends View{
public function index(){
$mysidia = Registry::get("mysidia");
$home = SCRIPTPATH. "/";
$pagination = $this->getField("pagination");
$news = $this->getField("news");
$document = $this->document;
$document->setTitle("News");
$document->add(new Comment("<div id='news' class='news'>"));
$iterator = $news->iterator();
while($iterator->hasNext()){
$news = $iterator->next();
$newsField = new Fieldset("news");
$comments = $news->getCommentNumber();
$newsField->add(new Comment("<span class='date'>Date: {$news->getDate()}</span><br>",FALSE));
$newsField->add(new Comment("<span class='title'><a href='{$home}news/view/{$news->getID()}'>{$news->getTitle()}</span>",FALSE));
$newsField->add(new Comment("<span class='author'>by <a href='{$home}profile/view/{$news->getUsername()}' target='_blank'>{$news->getUsername()}</a></span>",FALSE));
$newsField->add(new Comment("<span class='comment'><a href='{$home}news/view/{$news->getID()}'>Comments({$comments})</a></span><br>",FALSE));
$newsField->add(new Comment("<span class='content'><img class='authorimg' src='{$home}{$news->getProfile()->getAvatar()}'>{$news->getContent()}</span>",FALSE));
$document->add($newsField);
}
$document->add(new Comment('</div>'));
$document->addLangvar($pagination->showPage());
}
public function view(){
$mysidia = Registry::get("mysidia");
$home = SCRIPTPATH. "/";
$document = $this->document;
$news = $this->getField("news");
$comments = $this->getField("newscomments");
$pagination = $this->getField("pagination");
($news->getPosted() == "yes")? $newsState = "":$newsState = " (draft)";
$document->setTitle("news");
$document->add(new Comment("<div id='news' class='news'>"));
$newsField = new Fieldset("news");
$newsField->add(new Comment("<span class='date'>Date: {$news->getDate()}</span><br>",FALSE));
$newsField->add(new Comment("<span class='title'>{$news->getTitle()}{$newsState}</span>",FALSE));
$newsField->add(new Comment("<span class='author'>by <a href='{$home}profile/view/{$news->getUserID()}' target='_blank'>{$news->getUsername()}</a></span>",FALSE));
$newsField->add(new Comment("<span class='content'><p><img class='authorimg' src='{$home}{$news->getProfile()->getAvatar()}'>{$news->getContent()}<p></span>",FALSE));
$document->add($newsField);
$document->add(new Comment("<h1>comments</h1>",FALSE));
if($mysidia->input->post("submit")){
$date = $news->todayDate();
$content = $mysidia->input->rawPost("commenttext");
$user = $mysidia->user->getID();
$nid = $news->getID();
$news->addComment($content,$date,$user);
Header('Location: ' . $_SERVER['REQUEST_URI']);
}
$count = $mysidia->db->select("newscomments",["cid"],"nid = {$news->getId()}")->rowCount();
if($count == 0){
$document->add(new Comment("There are no comments to display."));
}
else{
$iterator = $comments->iterator();
while($iterator->hasNext()){
$comments = $iterator->next();
$commentField = new Fieldset("comment");
$commentField->add(new Comment("<span class='cdate'>Date: {$comments->getDate()}</span><br>",FALSE));
$commentField->add(new Comment("<span class='cauthor'>by <a href='{$home}profile/view/{$comments->getUserID()}' target='_blank'>{$comments->getUsername()}</a></span>",FALSE));
$commentField->add(new Comment("<span class='ccontent'><p><img src='{$home}{$comments->getProfile()->getAvatar()}'>{$comments->getComment()}<p></span>",FALSE));
$document->add($commentField);
}
$document->addLangvar($pagination->showPage());
}
if($news->getAllowComment() == "yes"){
$editor = new CKEditorBuilder("basic");
$commentForm = new Form("comment", "", "post");
$commentForm->add(new Comment("<br>Leave a comment below", FALSE));
$commentForm->add($editor->buildEditor("commenttext", "CKEditor for Mysidia " . Mysidia::version));
$commentForm->add(new Button("Comment", "submit", "submit"));
$document->add($commentForm);
}
else{
$document->add(new Comment("<br>Comments have been disabled.</div>",FALSE));
}
}
}
?>
That should allow you to view news on your site, read/add comments, etc. Now we need to add AdminCP functions!
Attachments
Last edited: