First, we need to add the following columns to the [prefix]_users table:
Finally, go to view/main and create a file called hiloview.php. Paste the following code:
This is my first time making a mod/addon so if you have any trouble with it or can think of a way to do it better, I'm here!!~
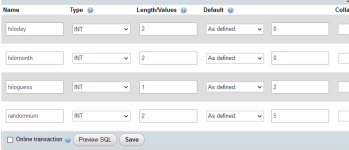
- hiloday and hilomonth will be a two digit representation of the day and month a player last played the game. (I included both so if they go exactly a month without playing, it won't mess up on them. You can also add a hiloyear but I thought that to be overkill.....)
- hiloguess should be a single digit integer. It's default value should be one less than you intend to give guesses. I give 3 guesses, so my default value is 2.
- randomnum is going to be changing every time a player plays. My number guessing game goes from 1-10, so I only need a 2 digit integer. I set it at 5 for the default, but like I said, it's going to be changing
PHP:
<?php
namespace Controller\Main;
use Resource\Core\AppController;
use Resource\Core\Registry;
class HiloController extends AppController {
public function __construct() {
parent::__construct("member");
}
public function index() {
$mysidia = Registry::get("mysidia");
}
}
?>
Finally, go to view/main and create a file called hiloview.php. Paste the following code:
PHP:
<?php
namespace View\Main;
use Controller\Main;
use Model\DomainModel\Member;
use Model\DomainModel\OwnedItem;
use Model\DomainModel\Item;
use Resource\Core\Registry;
use Resource\Core\View;
use Resource\GUI\Document\Comment;
use Resource\GUI\Component\Link;
class HiloView extends View
{
public function index()
{
$mysidia = Registry::get("mysidia");
$document = $this->document;
$thisMonth = date("m");
$today = date("d");
$number = $_POST['number_entered'];
$submitbutton = $_POST['submit'];
$randomnumber = rand(1,10);
$guessesLeft = $mysidia->user->hiloguess;
$document->setTitle("Higher or Lower??");
//this only lets players play once per day. at the end of the code, it sets hiloday and hilomonth to today's date.
if (($mysidia->user->hiloday) != $today || ($mysidia->user->hilomonth) != $thisMonth) {
$document->add(new Comment("
<form action='' method='POST'>
Guess a number between 1 and 10!
<input type='text' name='number_entered' value=''/><br><br>
Result:
"));
if (!empty($submitbutton) && !empty($number)) {
if (($number > 0) && ($number < 11)) {
if (($number != ($mysidia->user->randomnum)) && ($guessesLeft == 0)) {
$document->add(new Comment('Incorrect guess. The correct number was ', FALSE));
$document->add(new Comment(($mysidia->user->randomnum), FALSE));
$document->add(new Comment('. <br>Don\'t worry, you still get some a gold medal...'));
$mysidia->db->update(
"users",
[
"hiloday" => $today,
"hilomonth" => $thisMonth,
],
"username='{$mysidia->user->getUsername()}'"
);
$mysidia->db->update(
"users",
["hiloguess"=>2,
"randomnum"=>$randomnumber,],
"username='{$mysidia->user->getUsername()}'"
);
//change the item name to an item in your database to award it as a prize.
$ownedItem = new OwnedItem("Medal", $mysidia->user->getID());
$trueItem = new OwnedItem($ownedItem->getItemID(), $mysidia->user->getID());
$trueItem->add(1, $mysidia->user->getID());
//if they're not out of guesses, it will tell them if they need to guess higher or lower.
} elseif (($number != ($mysidia->user->randomnum)) && ($guessesLeft != 0)) {
if ($number > ($mysidia->user->randomnum)) {
$document->add(new Comment('Incorrect. My number is lower. Try again.'));
} else {
$document->add(new Comment("Incorrect. My number is higher. Try again."));
}
$mysidia->db->update(
"users",
["hiloguess" => ($guessesLeft -= 1)],
"username='{$mysidia->user->getUsername()}'"
);
$document->add(new Comment("You have ", FALSE));
$document->add(new Comment($guessesLeft, FALSE));
$document->add(new Comment(" guess(es) left."));
} else {
$document->add(new Comment(($mysidia->user->randomnum), FALSE));
$document->add(new Comment(' is correct! You got it right. You get the grand prize! Check your inventory!'));
$mysidia->db->update(
"users",
[
"hiloday" => $today,
"hilomonth" => $thisMonth,
],
"username='{$mysidia->user->getUsername()}'"
);
$mysidia->db->update(
"users",
["hiloguess"=>2,
"randomnum"=>$randomnumber,],
"username='{$mysidia->user->getUsername()}'"
);
//again, update the item name to one in your site.
$ownedItem = new OwnedItem("Gem", $mysidia->user->getID());
$trueItem = new OwnedItem($ownedItem->getItemID(), $mysidia->user->getID());
$trueItem->add(1, $mysidia->user->getID());
}
}
}
$document->add(new Comment('
<br><br>
<input type="submit" name="submit" value="Guess"/><br><br>
</form>
'));
} else {
//this resets their random number again, but still won't let them play until the next day
$document->add(new Comment("You've already played today. Come back tomorrow!"));
$mysidia->db->update(
"users",
["hiloguess"=>2,
"randomnum"=>$randomnumber],
"username='{$mysidia->user->getUsername()}'"
);
}
}
}
This is my first time making a mod/addon so if you have any trouble with it or can think of a way to do it better, I'm here!!~